Python is a versatile and user-friendly programming language, and understanding the differences between lists and tuples is crucial for efficient programming. Lists and tuples are both collections of items, but they differ in terms of mutability, performance, and usage. In this article, we will explore the difference between list and tuple in python and cover practical examples to help you understand their behavior.
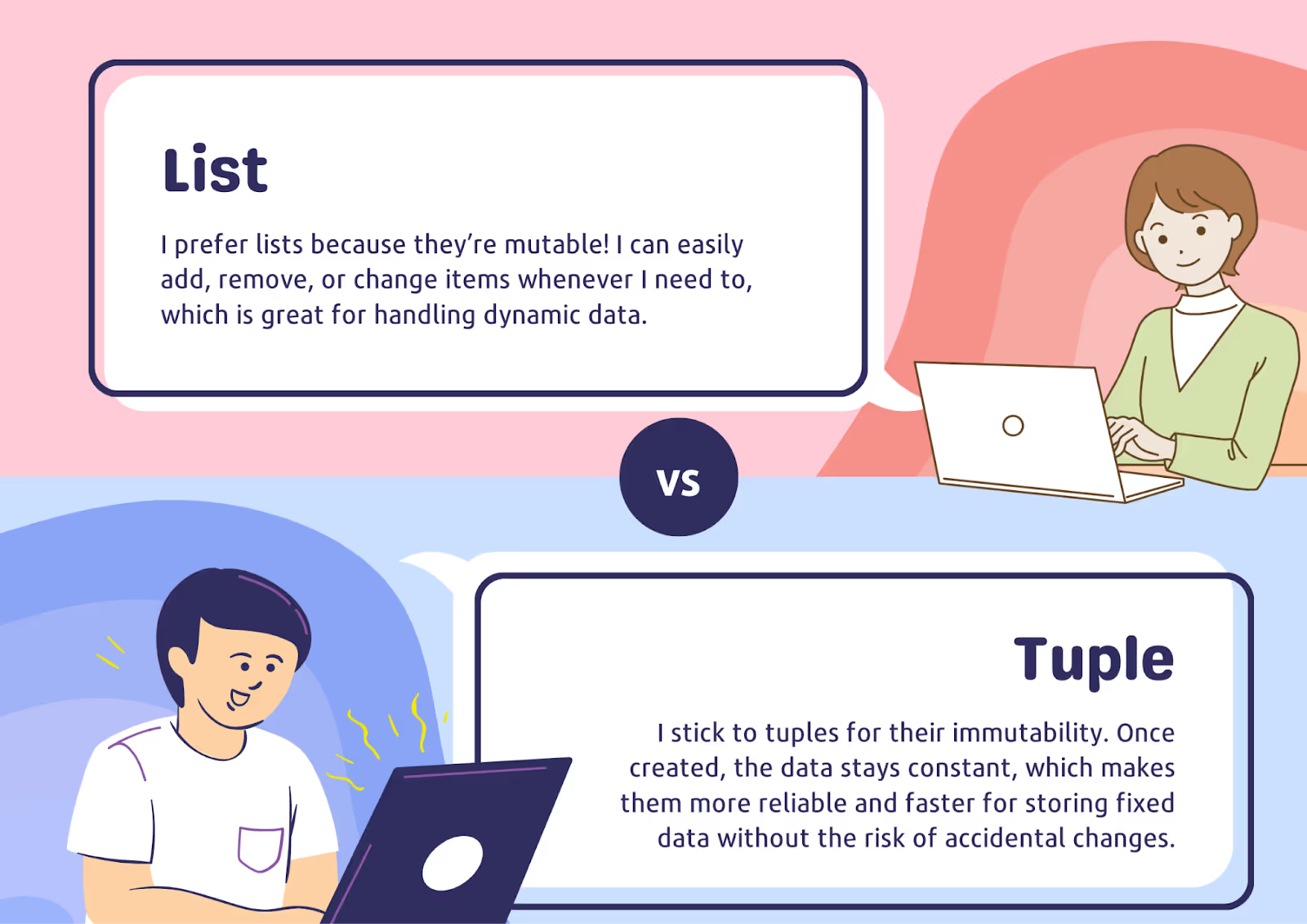
What Is a List in Python?
A list in Python is a mutable (modifiable) data structure used to store a collection of items. It is one of the most commonly used data types due to its flexibility and ease of use.
Why Are Lists Important? Lists are essential in Python programming because they provide a dynamic structure for handling data. They are widely used in algorithms and data processing tasks, especially in data science, where they can be used to store intermediate results, datasets, or features.
Characteristics of Lists:
- Mutable: You can modify the contents of a list (e.g., adding, updating, or removing elements).
- Ordered: Lists maintain the order of elements, meaning the items will appear in the same sequence as they were added.
- Dynamic: Lists can grow or shrink in size depending on the operations performed on them.
- Allows Duplicates: Lists can contain duplicate items.
List Syntax:
my_list = [1, 2, 3, 4, "Python", True]
Common List Methods:
- append(): Adds an element to the end of the list.
- remove(): Removes a specific element.
- pop(): Removes and returns the last element.
- sort(): Sorts the list in ascending order.
- reverse(): Reverses the order of elements.
Example:
my_list = [1, 2, 3, 4]
my_list.append(5) # Adds an element to the list
my_list.remove(2) # Removes the element 2
my_list.sort() # Sorts the list
print(my_list) # Output: [1, 3, 4, 5]
What Is a Tuple in Python?
A tuple in Python is an immutable (non-modifiable) data structure. It is typically used to store a collection of items that should remain constant throughout the program.
Why Are Tuples Important? Tuples are valuable when data should remain unchanged. They are useful in scenarios where the integrity of data is crucial, such as passing data between functions or working with fixed collections. In data science, tuples can be used to represent constant parameters, fixed data, and feature sets.
Characteristics of Tuples:
- Immutable: Once a tuple is created, its contents cannot be modified.
- Ordered: Like lists, tuples maintain the order of elements.
- Static Size: Since tuples are immutable, their size remains constant after creation.
- Allows Duplicates: Tuples can contain duplicate items.
Tuple Syntax:
my_tuple = (1, 2, 3, 4, "Python", True)
Common Tuple Methods:
- count(): Counts the occurrences of a specific element.
- index(): Returns the index of a specific element.
Example:
my_tuple = (1, 2, 3, 4)
print(my_tuple.count(2)) # Output: 1 (Counts occurrences of 2)
print(my_tuple.index(3)) # Output: 2 (Index of element 3)
Key Differences Between List and Tuple
Let's compare lists and tuples based on mutability, performance, syntax, and usage cases:
- Mutability:
some text- List: Mutable; you can change its contents.
- Tuple: Immutable; once created, its contents cannot be modified.
- Performance:
some text- List: Slower because Python must handle changes to the list.
- Tuple: Faster because they are optimized for read-only access.
- Syntax:
some text- List: Defined using square brackets [].
- Tuple: Defined using parentheses ().
- Use Cases:
some text- List: Used when the data may change during the program (e.g., storing user inputs).
- Tuple: Used when you want to keep data constant (e.g., fixed parameters or datasets that should remain unchanged).
- Importance in Data Science:
some text- In data science, lists are useful for tasks like feature engineering and iterative processing of datasets.
- Tuples are ideal for fixed data structures such as feature labels and constant parameters.

Example Highlighting Differences:
# List Example
my_list = [1, 2, 3]
my_list[0] = 10 # Allowed
print(my_list) # Output: [10, 2, 3]
# Tuple Example
my_tuple = (1, 2, 3)
# my_tuple[0] = 10 # Raises a TypeError
print(my_tuple) # Output: (1, 2, 3)
Detailed Summary of Differences:
Here’s a table summarizing the key differences between lists and tuples:
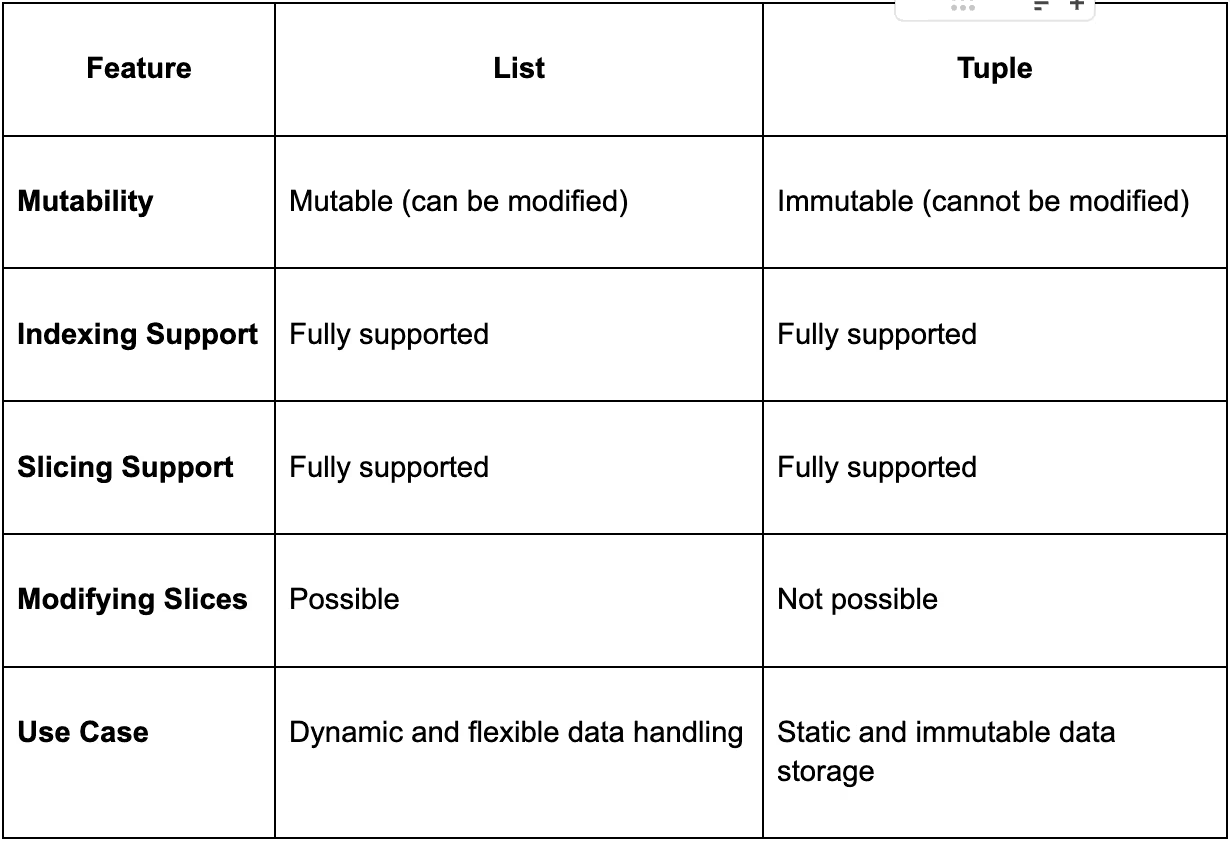
Manipulating Lists and Tuples
List Manipulations:
- Adding Elements:
some text- append() adds an element to the end of the list.
- insert() adds an element at a specified index.
- Removing Elements:
some text- remove() removes a specific element.
- pop() removes and returns the element at a specified index.
- Sorting Elements:
some text- sort() sorts the list in place.
- Reversing Elements:
some text- reverse() reverses the order of elements.
- Copying a List:
some text- copy() creates a shallow copy of the list.
- clear() removes all elements from the list.
Tuple Manipulations:
Tuples are immutable, so methods like append(), remove(), or sort() cannot be used. You can, however, convert a tuple to a list, perform modifications, and then convert it back to a tuple.
Example List Operations:
# List Example
my_list = [10, 20, 30, 40]
# Append
my_list.append(50)
print("After append:", my_list)
# Insert
my_list.insert(2, 25)
print("After insert:", my_list)
# Remove
my_list.remove(30)
print("After remove:", my_list)
# Pop
popped_element = my_list.pop(3)
print("Popped element:", popped_element)
print("After pop:", my_list)
# Sort
my_list.sort()
print("After sort:", my_list)
# Reverse
my_list.reverse()
print("After reverse:", my_list)
# Index
index_of_20 = my_list.index(20)
print("Index of 20:", index_of_20)
# Count
count_of_20 = my_list.count(20)
print("Count of 20:", count_of_20)
# Copy
copy_list = my_list.copy()
print("Copy of list:", copy_list)
# Clear
my_list.clear()
print("After clear:", my_list)
Example Tuple Operations:
my_tuple = (10, 20, 30, 20)
# Index
index_of_20 = my_tuple.index(20)
print("Index of 20 in tuple:", index_of_20)
# Count
count_of_20 = my_tuple.count(20)
print("Count of 20 in tuple:", count_of_20)
Practical List and Tuple Creation:
- Lists creation in several ways:
some text- Using square brackets:
list1 = [1, 2, 3, 4] - Using the list() constructor:
list2 = list((5, 6, 7, 8)) - Using list comprehension:
squared_list = [x**2 for x in range(5)]
- Using square brackets:
- Tuples creation in different ways:
some text- Using parentheses:
tuple1 = (1, 2, 3, 4) - Using the tuple() constructor:
tuple2 = tuple([5, 6, 7, 8]) - Without parentheses (comma-separated values):
tuple3 = 9, 10, 11
- Using parentheses:
Interview Questions on Lists and Tuples
To help solidify your understanding of lists and tuples in Python, here are some interview questions you might encounter, along with answers and explanations.
- What is the main difference between a list and a tuple?
some text- Answer: A list is mutable, meaning you can modify its elements after it is created. A tuple, on the other hand, is immutable, meaning once it is created, its elements cannot be changed.
- Can you add or remove elements from a tuple?
some text- Answer: No, you cannot modify a tuple because it is immutable. You can, however, create a new tuple by concatenating other tuples or slicing them.
- What happens if you try to change an element of a tuple?
some text- Answer: If you try to modify an element of a tuple, it will raise a TypeError because tuples are immutable.
- How do you convert a list into a tuple?
some text- Answer: You can use the tuple() constructor to convert a list into a tuple.
my_list = [1, 2, 3]
my_tuple = tuple(my_list)
- What are some common use cases for tuples in Python?
some text- Answer: Tuples are often used for data that should not be modified, such as:some text
- Storing fixed configurations or settings.
- Returning multiple values from functions.
- Using tuples as dictionary keys (since tuples are hashable, unlike lists).
- Answer: Tuples are often used for data that should not be modified, such as:some text
- What is a potential advantage of using tuples over lists in terms of performance?
some text- Answer: Tuples are generally faster than lists for iteration and access because they are immutable, making them more optimized for read-only operations. Lists, being mutable, require additional memory management.
- How can you concatenate two tuples or two lists?
some text- Answer: You can concatenate lists and tuples using the + operator.
# List concatenation
list1 = [1, 2]
list2 = [3, 4]
concatenated_list = list1 + list2 # Output: [1, 2, 3, 4]
# Tuple concatenation
tuple1 = (1, 2)
tuple2 = (3, 4)
concatenated_tuple = tuple1 + tuple2 # Output: (1, 2, 3, 4)
- How can you remove duplicates from a list or tuple?
some text- Answer:some text
- List: You can use the set() function to remove duplicates and convert it back to a list.
- Answer:some text
my_list = [1, 2, 3, 1, 2]
unique_list = list(set(my_list)) # Output: [1, 2, 3]
some text- Tuple: Since tuples are immutable, you can first convert them to a set and then back to a tuple.
my_tuple = (1, 2, 3, 1, 2)
Unique_tuple= tuple(set(my_tuple)) # Output: (1, 2, 3)
Now you can learn the difference between list and tuple and dictionary in python along with other data structures and do the same kind of practice.
Conclusion
In summary, lists and tuples serve different purposes in Python. Lists are mutable and ideal for dynamic data handling, while tuples are immutable and suitable for storing constant data. By mastering the differences between these data structures and understanding when to use each, you can make your code more efficient and appropriate for various programming scenarios. Always choose the appropriate data structure depending on your need for mutability, performance, and data integrity.
By mastering lists and tuples, you can efficiently manage data in Python, whether you need to store dynamic collections or protect data integrity through immutability. Use lists for changeable data and tuples for fixed, unmodifiable data. Always select the data structure that fits your program’s needs!