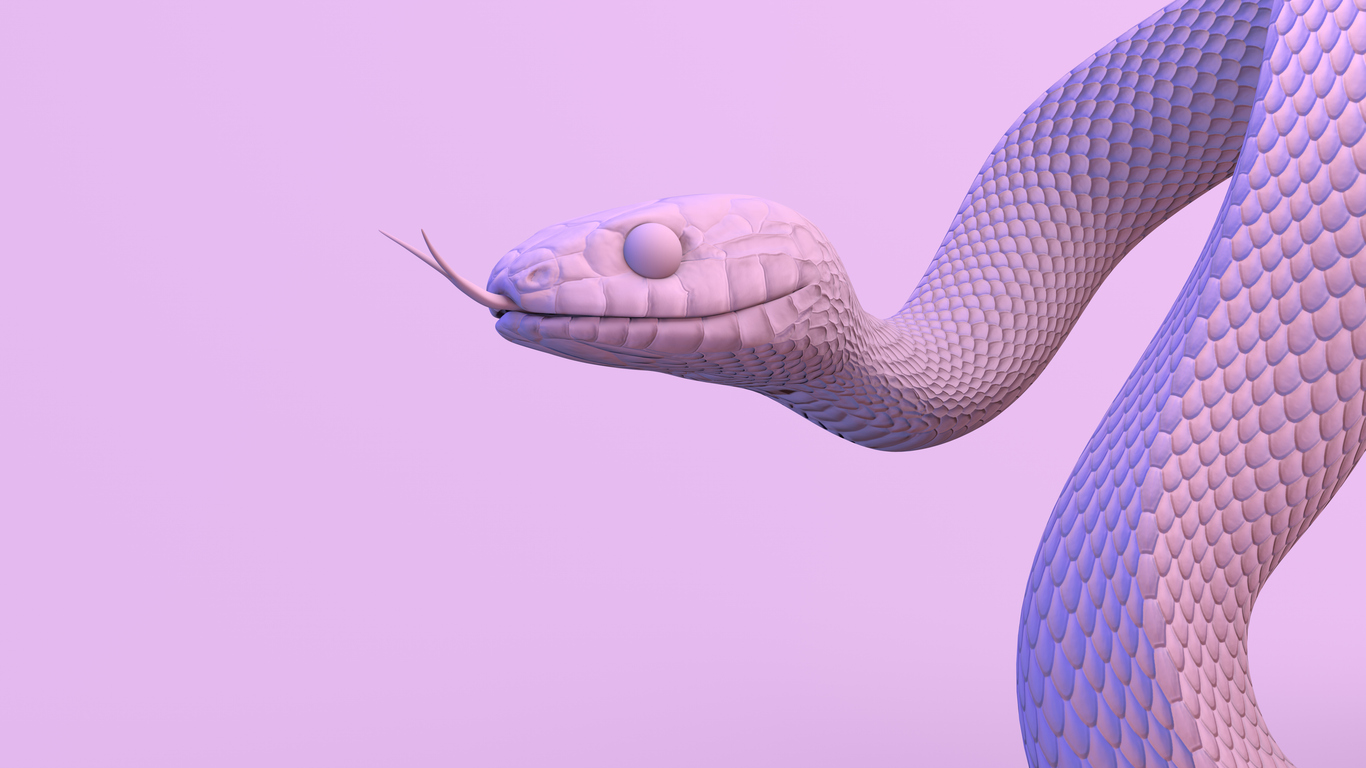
In any programming language, errors are inevitable. Whether it's due to a typo, wrong data type, or even an unexpected user input, handling errors effectively is crucial to building reliable software. Python provides robust features for exception handling, which allows developers to anticipate errors and manage them gracefully. This article will introduce exception handling, explain its importance, and demonstrate various techniques to handle exceptions efficiently.
What is Exception Handling in Python?
Exception handling is a mechanism that allows a program to detect and respond to runtime errors, also known as exceptions, without crashing the entire program. In programming, errors can occur for various reasons such as invalid user input, incorrect assumptions, or external system failures (like network problems or file access issues). These errors can lead to abrupt program termination if not properly handled. Exception handling allows developers to manage these errors more effectively by "catching" them and providing a way to recover from them or display a meaningful error message.
In Python, exceptions are objects that represent errors in the program. When something goes wrong, Python raises an exception, which can be caught and handled using the try and except blocks. The primary goal of exception handling is to make sure that the program continues to run smoothly despite encountering unexpected issues.
The Importance of Exception Handling
Exception handling in Python is essential for several reasons:
- Program Stability:
Without exception handling, an error could crash the entire program, leading to a bad user experience. For instance, if the user inputs invalid data or attempts to open a non-existent file, the program could terminate abruptly. By handling exceptions, the program can gracefully recover from errors and continue running. - Better User Experience:
Exception handling provides a way to communicate with the user about the error. Instead of the program crashing, users can be given helpful feedback, such as "Invalid input" or "File not found," allowing them to correct the issue and try again. - Preventing Unnecessary Termination:
In some cases, errors might not be critical to the overall program flow. With exception handling, you can allow the program to proceed or switch to an alternative route, preventing unnecessary termination and enabling the program to still achieve its purpose. - Debugging and Logging:
Exception handling allows developers to catch errors and log them for later analysis. By logging the error details (such as the type of exception and the stack trace), developers can easily trace the root cause of the issue, which is valuable for debugging and improving the code. - Graceful Resource Cleanup:
The finally block is particularly useful for cleaning up resources, like closing files or database connections, regardless of whether an exception occurs. This helps in maintaining good resource management and avoiding memory leaks or locked resources.

How Python Try Except Works
In Python, exception handling is a critical aspect of writing robust and reliable code. The primary structure used for handling exceptions is the try and except block. The goal is to separate the code that might raise an exception from the rest of the code, allowing you to handle errors in a way that doesn’t cause the program to crash. Here’s a detailed breakdown of how this works:
1. The try Block:
The try block contains the code that you expect might raise an exception. This could be anything from mathematical operations, like dividing by zero, to file handling where the file might not exist. If no exception occurs, the code inside the try block executes as normal.
Example:
try:
number = int(input("Enter a number: "))
result = 10 / number
except ZeroDivisionError:
print("You can't divide by zero!")
except ValueError:
print("Invalid input. Please enter a valid number.")
- In this example:some text
- If the user enters a valid number, the division will happen without issues.
- If the user enters a non-numeric input, a ValueError will be raised, and the error message “Invalid input. Please enter a valid number.” will be displayed.
- If the user enters 0, a ZeroDivisionError will be raised, and the message "You can't divide by zero!" will be displayed.
How it works:
The try block executes the code inside it. If everything works without raising an exception, the program proceeds to the next part of the code. However, if an exception occurs, Python stops executing the code inside the try block and jumps to the corresponding except block.
2. The except Block:
The except block defines how to handle exceptions raised in the try block. You can specify the type of exception you want to catch, or use a generic except to catch all exceptions. This allows you to control what happens when an error occurs, rather than letting the program crash unexpectedly.
Example with specific exception types:
try:
number = int(input("Enter a number: "))
result = 10 / number
except ZeroDivisionError:
print("You can't divide by zero!")
except ValueError:
print("Invalid input. Please enter a valid number.")
How it works:
The program tries to execute the code in the try block. If a ZeroDivisionError is raised (e.g., the user enters 0), it will jump to the except ZeroDivisionError block. Similarly, if a ValueError occurs (e.g., the user enters non-numeric input), it will jump to the except ValueError block. If no exceptions occur, the program simply continues executing normally.
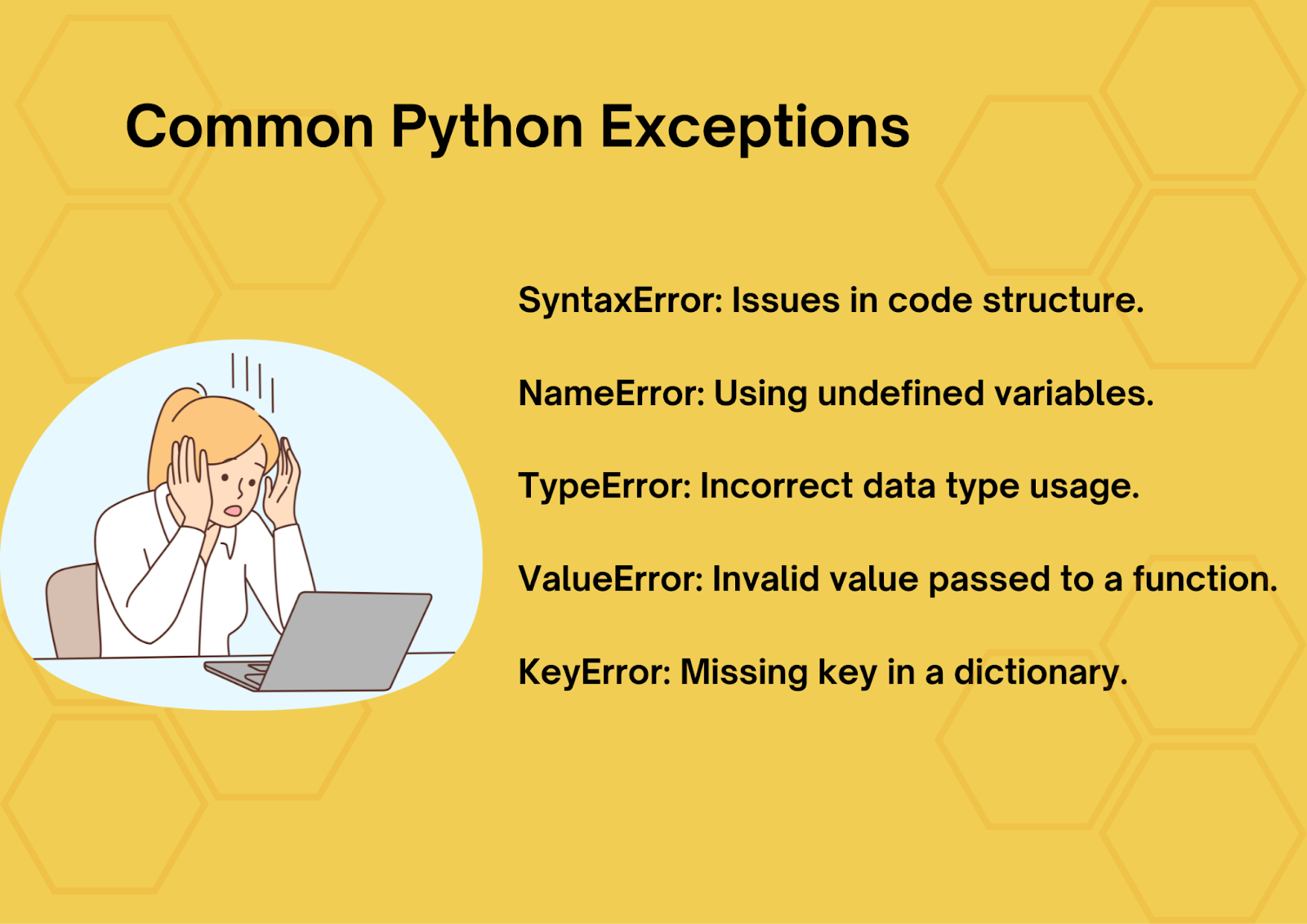
Python Catch Exception Techniques
While the basic try and except blocks are effective for catching exceptions, there are several advanced techniques that can make python catch exception techniques more robust and specific to your needs.
1. Catching Multiple Exceptions:
Sometimes, multiple exceptions might occur in the same block, and you might want to handle them in the same way. Python allows you to catch multiple exceptions in a single except block by listing them in a tuple. This is useful when you expect different types of errors but want to handle them with the same logic.
Example:
try:
number = int(input("Enter a number: "))
result = 10 / number
except (ZeroDivisionError, ValueError) as e:
print(f"An error occurred: {e}")
How it works:
If either a ZeroDivisionError or ValueError occurs, the exception will be caught by the except block. The variable e holds the error message, and it's printed out to inform the user of the issue. This method is efficient because it reduces code duplication.
2. Using the else Block:
The else block is an optional part of exception handling. It is executed only if no exceptions are raised in the try block. This is useful for executing code that should only run when the try block completes successfully.
Example:
try:
number = int(input("Enter a number: "))
result = 10 / number
except (ZeroDivisionError, ValueError) as e:
print(f"An error occurred: {e}")
else:
print(f"The result is {result}")
How it works:
If the try block runs without raising any exceptions (i.e., no error occurs), the code inside the else block is executed. In this case, the program will display the result of the division. If any exception occurs, the except block will handle it, and the else block will be skipped.
3. Using the finally Block:
The finally block is executed no matter what — whether an exception occurred or not. It is often used for cleanup activities like closing files, releasing network resources, or shutting down connections. It ensures that important tasks are performed regardless of whether the operation was successful or failed.
Example:
try:
file = open("data.txt", "r")
data = file.read()
except FileNotFoundError:
print("File not found.")
finally:
file.close() # Ensure the file is closed even if an error occurs
How it works:
Regardless of whether the file exists or not, the finally block will run, ensuring that the file is closed. This is crucial in resource management because it guarantees that system resources are released properly.
4. Custom Exception Handling:
Python allows you to create your own exceptions by defining a class that inherits from the built-in Exception class. Custom exceptions give you more control over the error handling process, especially when dealing with specific situations in your program.
Example:
class CustomError(Exception):
pass
try:
raise CustomError("Something went wrong!")
except CustomError as e:
print(f"Caught a custom error: {e}")
How it works:
In this example, a custom exception class CustomError is created. The try block raises this exception using the raise keyword, and the except block catches it, printing a message with the error. Custom exceptions are especially useful when dealing with domain-specific errors that don't fit into the built-in exception classes.
Why Should We Use Exception Handling?
Using python exception handling helps prevent your program from crashing due to unforeseen errors, making your code more robust. It also improves user experience, as users will receive clear feedback when something goes wrong. By catching and handling exceptions, you can identify, fix, and debug errors more effectively, ensuring a stable and reliable application.
When you catch exceptions using try except, you are not just preventing your program from failing, but you're also building an environment where the program can gracefully handle unexpected events. Python exception handling provides a clean way to handle errors without cluttering your code with unnecessary checks.
Conclusion
Exception handling is an essential technique for building robust, reliable, and user-friendly programs. It allows you to anticipate and respond to errors in a controlled manner, ensuring that your code continues running smoothly even when things go wrong. By using python try except else, and finally blocks, you can manage errors effectively, improve user experience, and make your Python programs more resilient and maintainable.
In the next section, we’ll dive deeper into specific techniques for catching exceptions, like catching multiple exceptions at once, and customizing exceptions for more fine-grained control. But for now, understanding how Python handles exceptions and why it matters is the foundation of writing good, error-resistant code.