Introduction
Let’s be honest—when you first hear the term "regular expressions" (or regex for short), it sounds like some cryptic spell from a wizarding school. But fear not, my Python-loving friend! What is a regular expression in Python, you ask? It’s actually one of the most powerful tools you can add to your programming toolbox. Think of them as the Swiss Army knife of text processing—they can find, match, replace, and transform text faster than you can say, "Wait, what’s a regex again?"
Simply put, regular expressions in Python are patterns you can use to search through text in a very precise way. Whether you’re hunting down misspelled words, extracting data from a messy file, or validating email addresses to make sure no one signs up as “definitelynot_a_bot@fakeemail.com,” regex has got your back. And the best part? Python makes working with regex relatively painless—emphasis on "relatively."
In this article, we’re going to break down regex concepts step-by-step. By the end, you’ll not only understand what is regular expression in Python, but you’ll also learn about types of regular expression in Python and how to use them effectively with plenty of useful regular expression in Python example snippets. Don’t worry—we’ll keep things simple, fun, and way less scary than they sound. Ready to unlock the mysteries of regex? Let’s roll!
RegEx Syntax
A regular expression make appear complex at first glance, but we can make it easier by breaking it down:

This is an example of a regular expression. The syntax is

RegEx Patterns
Think of RegEx patterns as special patterns you can create to describe things you’re looking for in text. The components of RegEx patterns are:
- Literals:
These are the plain letters and numbers you type that match exactly what you type in your pattern. If you search for cat, it will match any place it finds "cat" in the text.
- Wildcards:
- . (dot): This is a wildcard that matches any single character, except a newline. So, c.t would match "cat", "cot", "cut", etc.
- ^ and $: These symbols represent the start and end of a line.^Hello finds "Hello" only if it’s at the start of the line. world$ finds "world" only if it’s at the end of the line.
- \b – Word boundary (useful for matching whole words).
- \B – Non-word boundary.
- Character Sets and Ranges
- [] (square brackets): This is like a menu of characters to choose from. [aeiou] matches any one vowel, and [123] matches "1", "2", or "3". You can also define ranges inside brackets:
[a-z] matches any lowercase letter.
[0-9] matches any digit from 0 to 9.
- [^ ] (caret inside brackets): The caret inside brackets means "NOT". So, [^0-9] matches any character that’s not a digit.
- Repetitions
- *: Matches zero or more of the previous character or group. So, ba* matches "b", "ba", "baa", "baaa", etc.
- +: Matches one or more of the previous character or group. So, ba+ matches "ba", "baa", "baaa", etc., but not "b" by itself.
- ?: Matches zero or one of the previous character or group, which means it’s optional. colou?r matches both "color" and "colour".
- {n}: Matches exactly n times. a{3} will match "aaa" but not "aa" or "aaaa".
- {n,}: Matches n or more times. a{2,} will match "aa", "aaa", "aaaa", etc.
- {n,m}: Matches between n and m times. a{2,4} will match "aa", "aaa", and "aaaa", but not "a" or "aaaaa".
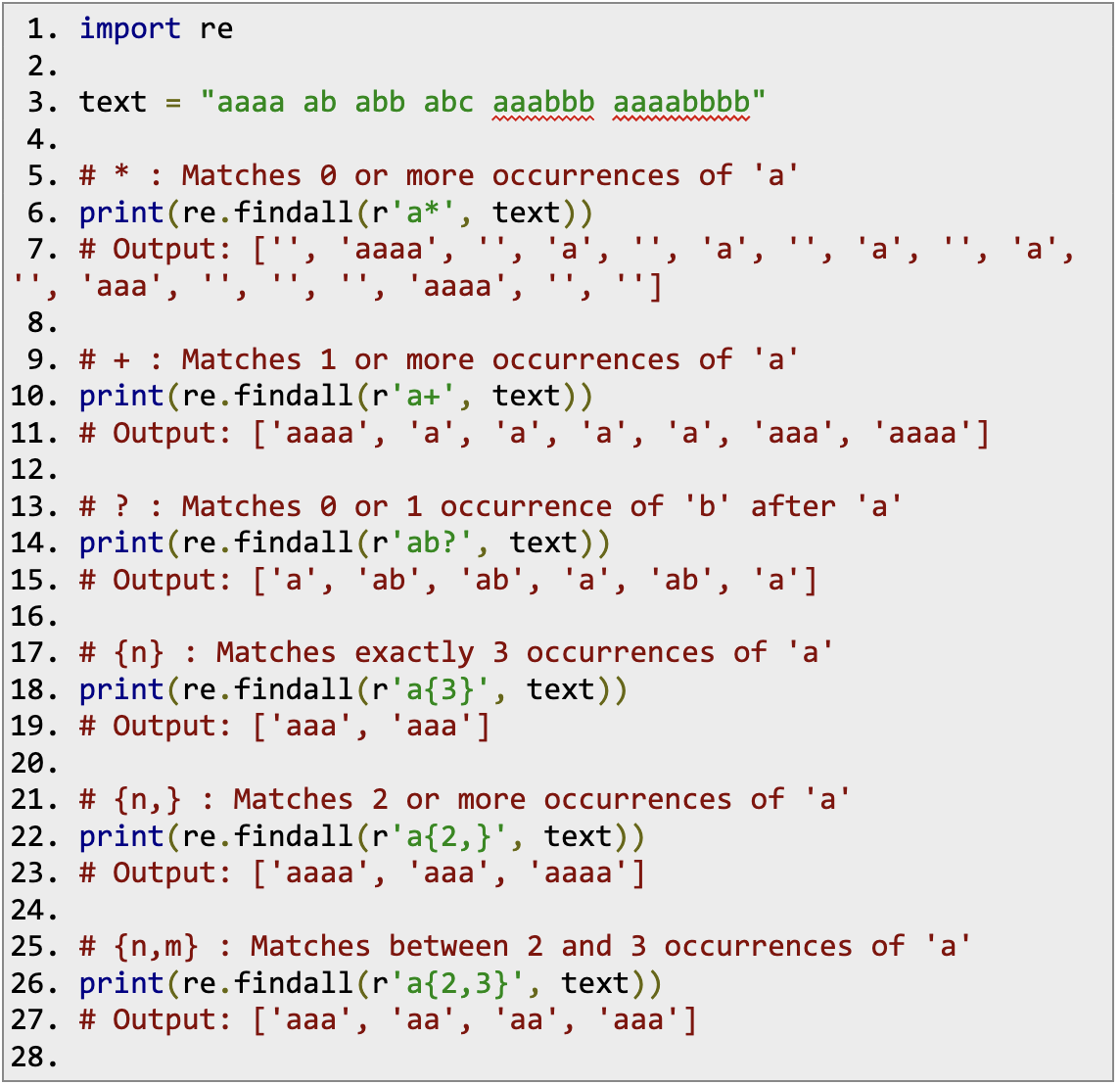
- Escaping Special Characters
If you want to match a character that’s usually a special symbol (like . or *), you need to use a backslash \ to escape it. For example, to search for a literal dot, you’d write \. instead of a single dot .
- Shorthand Character Classes
- \d: Matches any digit (same as [0-9]).
- \D: Matches any non-digit character (same as [^0-9]).
- \w: Matches any word character, which includes letters, numbers, and underscores (same as [a-zA-Z0-9_]).
- \W: Matches any non-word character (anything that isn’t \w).
- \s: Matches any whitespace character (spaces, tabs, line breaks).
- \S: Matches any non-whitespace character.
- Groups and Alternations
- () (parentheses): These are used to create groups. Grouping is helpful for finding or repeating parts of the pattern together. For example, (ab)+ would match "ab", "abab", "ababab", etc.
- | (pipe): This works like an "or". So, cat|dog matches either "cat" or "dog".
- Lookahead and lookbehind assertions
Lookaheads are like saying, "Check what’s coming next before deciding to match something." They peek ahead but don’t include what they see in the match. Similarly, lookbehinds are like saying, "Check what’s behind before deciding to match something." They peek backward but don’t include what they see in the match.
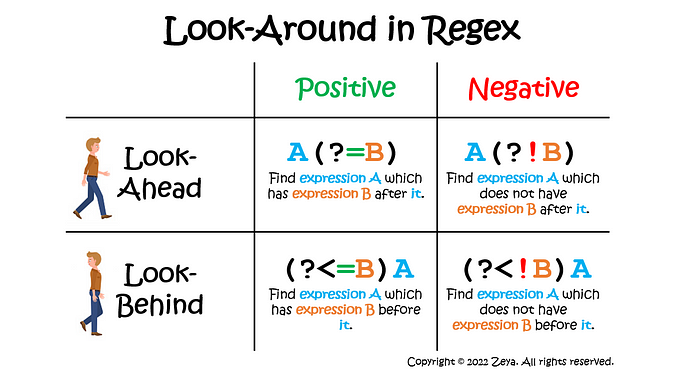
RegEx Functions
The re module offers a set of functions that allows us to search a string for a match. Before that, let’s look at some of the components of regex functions:
- Patterns (see above): The regex expression (a sequence of characters) used to define the search criteria.
- Match: A successful instance where the regex pattern has found a part of the string that satisfies the defined criteria.
- Flags: Modifiers that change how your pattern behaves when matching text. Here are some of the most commonly used flags: some text
- re.IGNORECASE (re.I)– Tells Python to ignore the differences between upper and lower case
- re.MULTILINE (re.M)- Changes how ^ (start of the string) and $ (end of the string) work. Normally, these match only the start and end of the entire string, but with this flag, they match at the start and end of each line in a multi-line string.
- re.DOTALL (re.S) – Makes the . (dot) match everything, including line breaks. Normally, . matches any character except line breaks.
- re.VERBOSE (re.X) - Lets you write regex patterns in a more readable way by allowing spaces and comments inside the pattern. Here is an example:
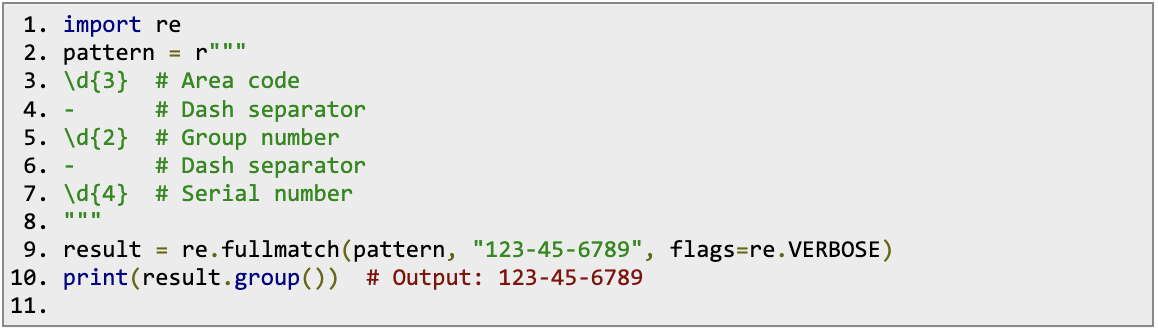
- Match Objects: An object returned by a function if a match is found. It contains information about the match, such as the matched string and its position in the text. Match objects can be used with methods which returns specific parts of the match objects. Here are common methods you can use with match objects:some text
- .group() – Returns the part of the string matched by the regular expression.
- .start() – Returns the start index of the match in the original string. Useful when you need to know where the match starts.
- .end() - Returns the end index of the match (the position just after the last matched character). Can be helpful when you need the range of the matched string.
- .span() - Returns a tuple (start, end) giving the start and end positions of the match.
- .groups() - Returns a tuple of all captured groups in the pattern (if any). A captured group is a part of the match enclosed in parentheses ().
- .group(n) - Returns the n-th captured group. If n is 0, it returns the entire match.
- .expand() - String with groups expanded using a format string.
- Group: A part of a regex pattern enclosed in parentheses () that allows you to capture a specific portion of the string that matches that part of the pattern. Here is an example of using the group(n) method:

- Backreference: Refers to a previously captured group in the same pattern. Backreferences are used by \1, \2, etc., where the number corresponds to the group number.
re.match()
Checks for a match only at the beginning of a string. Returns a match object if found, otherwise None

re.search()
Checks for a match anywhere in the string, and returns the first match object if found, otherwise None

re.findall()
Checks for matches in the string, and returns a list of match objects if found, otherwise None

re.split()
Splits strings by the matches of the pattern. Returns a list of strings. Maxsplit determines the maximum number of splits to be made.

re.fullmatch()
Checks if the entire string matches the pattern. Returns the match object if true, otherwise None

re.compile()
Compiles a regex pattern into a regex object, essentially adding an alias to the pattern which is useful for repeated matches.

Here is an example:

re.escape()
Escapes all special characters in a string so that they are treated as literals in the pattern. This is useful when you want to use a string that might contain characters that have special meaning in regular expressions (like ., *, +, ?, [], etc.) but you want to match the string exactly as it is, without those special meanings being applied. This method is almost entirely used as part of the search pattern. For example:

But it’s unlikely you’re looking for that output. However, if you use it in conjunction with other functions:

You get the output exactly as expected.
re.finditer()
This function finds all matches of a pattern in a string, but instead of just a list, it returns an iterable object, which tells you where the match is on a string. Here’s a simple example:
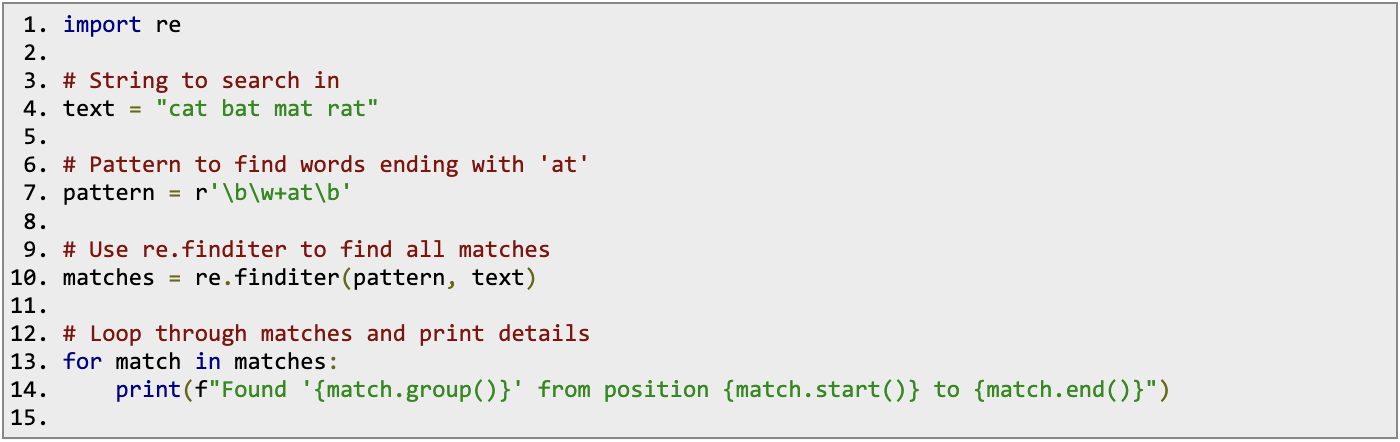
Matches is used to store the iterable object (the output of re.finditer(pattern, text)).
for match in matches loops through each match and prints:
- The matched text using match.group()
- The position of the match using match.start() and match.end()
The output will be:

Conclusion
And there you have it, folks—your crash course in the wonderful, sometimes weird, world of regular expressions in Python! We covered everything from what is regular expression in Python to types of regular expression in Python, along with plenty of regular expression in Python examples to help you on your way.
Regular expressions can save you loads of time when you're working with text data—whether you’re cleaning messy datasets, validating user input, or searching for patterns faster than you can say “Ctrl+F.” Sure, they look like alien code at first, but the more you use them, the more you'll realize they’re less like aliens and more like super-powered sidekicks.
The key to mastering regex is practice, so don’t be afraid to get your hands dirty. Fire up that Python shell and experiment! Break stuff, see what works, and don’t worry if you mess up a few times—that’s how you learn. And hey, if all else fails, remember that Google loves regex questions (trust us on this).