Whether you're a beginner learning your first programming language or an experienced developer preparing for advanced technical interviews, practicing Python programs daily is the key to mastering coding skills.
Python’s clean syntax, extensive library support, and readability make it one of the most beginner-friendly and interview-ready languages out there. From simple tasks like reversing a string to solving complex algorithmic challenges like graph traversals or dynamic programming problems—Python has your back.
In this article, we’ll walk you through a curated list of the top python programs for practice, starting from basic python programs for practice and gradually progressing to python programming questions for practice that will help you crack coding rounds, technical interviews, and competitive programming challenges.
Whether you want to become a confident Python programmer or ace your next coding round, this guide is for you.
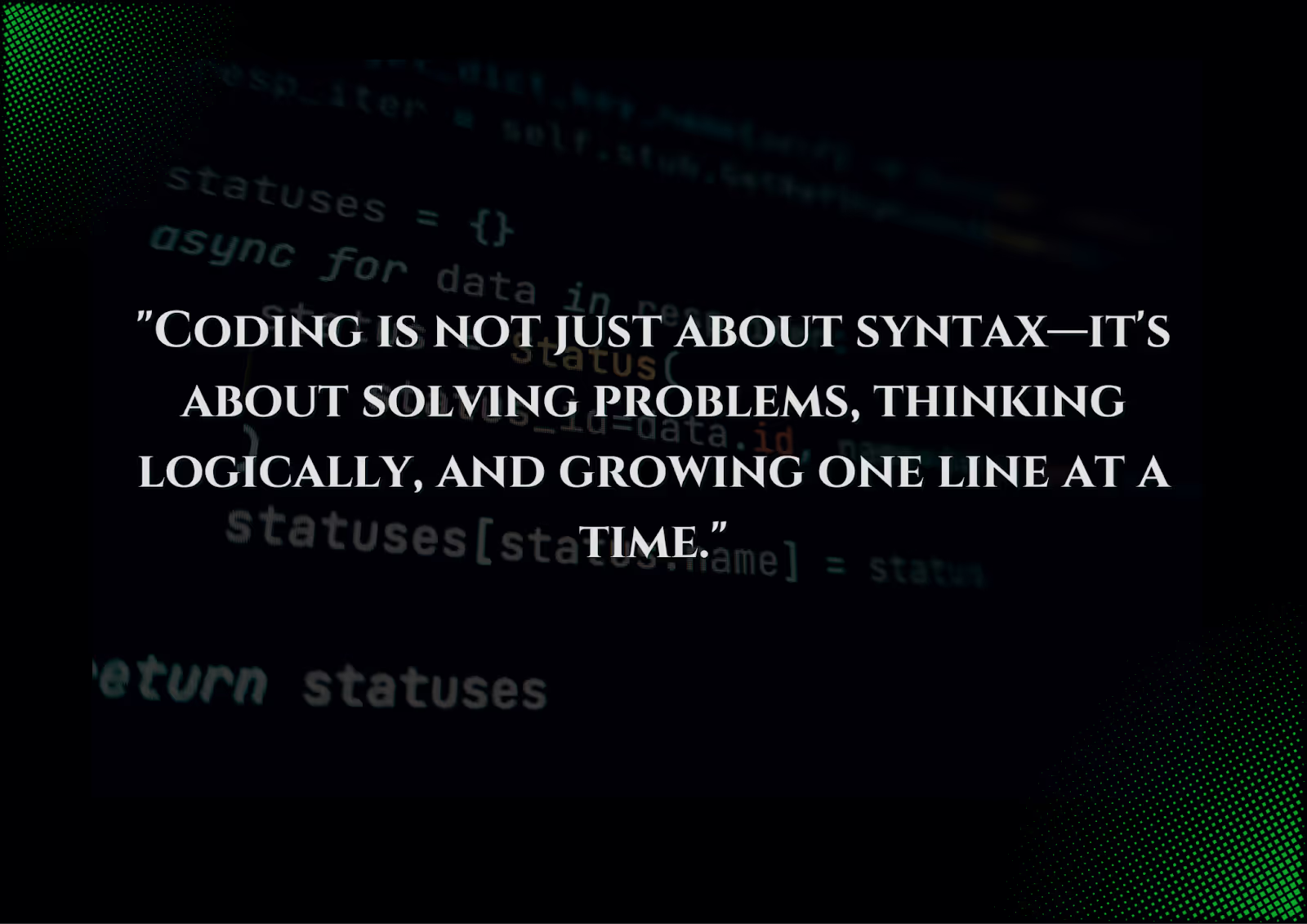
Setting Up for Success
Before diving into Python programs for practice, make sure your environment is ready:
Tools You Can Use:
- VS Code or PyCharm (for full project development)
- Jupyter Notebook (great for visual and interactive learning)
- Online Editors: Replit, Programiz, Google Colab
Learning Approach That Works:
- Understand the problem: Don’t rush. Read the problem twice.
- Plan your logic: Use flowcharts or pseudocode if needed.
- Code your solution: Focus on writing clean, readable Python.
- Test and debug: Check edge cases.
- Optimize: Try reducing time and space complexity.
Now that your setup is ready, let’s move to the actual coding part!
Basic Python Programs for Practice
This section is all about getting your fundamentals right. These basic python programs for practice are perfect if you’re just starting out or want to brush up your core logic-building skills.
Simple Input/Output Programs
- Print “Hello, World!”
- Take user input and print it
- Swap two variables without a third variable
Math-Based Problems
- Find factorial of a number
- Generate Fibonacci series up to ‘n’ terms
- Check if a number is prime
- Armstrong number checker
- Find GCD and LCM of two numbers
Number & Digit Problems
- Sum of digits of a number
- Reverse a number
- Check if a number is a palindrome
- Count digits, even/odd digits in a number
Pattern Printing
- Right-angle triangle using *
- Number pyramid
- Diamond shape using characters
- Floyd’s triangle
String Basics
- Check if a string is a palindrome
- Count vowels and consonants
- Reverse a string without using slicing
- Remove duplicate characters
Mastering these will help you build the confidence to take on more complex python programming questions.
Intermediate Python Programs for Practice
Now that you’re comfortable with basic problems, it’s time to level up. These intermediate python programs for practice will strengthen your understanding of data structures, string operations, and file handling—all crucial for real-world applications and coding interviews.
String Manipulation Programs
- Check if two strings are anagrams
Example: "listen" and "silent" → True - Find the first non-repeating character in a string
- Count the frequency of each character Use collections.Counter or a manual dictionary.
- Capitalize the first letter of each word in a sentence
- Remove all special characters from a string
List and Dictionary Programs
- Remove duplicates from a list
- Find the second largest number in a list
- Merge two sorted lists
- Reverse a list without using built-in functions
- Perform CRUD operations using a dictionary (Create, Read, Update, Delete key-value pairs)
File Handling in Python
- Read a text file and count words, lines, and characters
- Copy the contents of one file to another
- Find and replace text in a file
- Append text to an existing file
Basic Algorithm Practice
- Bubble sort, selection sort, insertion sort
- Linear and binary search
- Find the missing number in a list from 1 to N
- Check if a list is sorted
Recursion-Based Problems
- Calculate factorial using recursion
- Fibonacci using recursion
- Sum of digits using recursion
- Check palindrome using recursion
Advanced Python Programming Questions for Practice
This section is your prep ground for coding interviews, product-based company tests, and competitive programming. These python programming questions for practice demand a good grasp of algorithms, data structures, and Python’s built-in capabilities.
Popular Coding Interview Questions (with Concepts)
1. Two Sum Problem
Find two numbers in a list that add up to a target.
Concept: Hashing
def two_sum(nums, target):
seen = {}
for i, num in enumerate(nums):
complement = target - num
if complement in seen:
return [seen[complement], i]
seen[num] = i
2. Longest Substring Without Repeating Characters
Concept: Sliding Window, HashSet
3. Merge Intervals
Concept: Sorting, Greedy Algorithm
Use for scheduling problems or overlapping intervals.
4. Reverse a Linked List
Concept: Pointers, Iterative or Recursive
Create a custom LinkedList class if needed.
5. Detect Cycle in a Linked List
Concept: Floyd’s Cycle Detection (Tortoise and Hare)
6. Check Balanced Parentheses
Concept: Stack
def is_balanced(expr):
stack = []
pairs = {')':'(', '}':'{', ']':'['}
for char in expr:
if char in '({[':
stack.append(char)
elif char in ')}]':
if not stack or stack.pop() != pairs[char]:
return False
return not stack
7. Find All Duplicates in a List (Without Extra Space)
Concept: Index Mapping, Sign flipping (for range 1 to N problems)
8. Search in Rotated Sorted Array
Concept: Modified Binary Search
Tested in many FAANG interviews.
9. LRU Cache Implementation
Concept: HashMap + Doubly Linked List
Can also be implemented using OrderedDict from collections.
10. Dijkstra’s Algorithm
Concept: Graphs, Priority Queue (heapq in Python)
Ideal for shortest path problems.
Additional Advanced Practice Ideas:
- Implement Trie (Prefix Tree)
- N-Queens Problem (Backtracking)
- Sudoku Solver
- Topological Sort (Graph – DFS or Kahn’s Algo)
- Dynamic Programming:
- Longest Increasing Subsequence
- 0/1 Knapsack
- Edit Distance
- Coin Change
- Longest Increasing Subsequence
Core Data Structures and Algorithms in Python
Understanding how data structures work internally is a must for advanced interviews and writing efficient code. This section will guide you to implement key data structures from scratch and solve classic problems using them.
These are must-do python programs for practice if you're preparing for system design or algorithm-intensive roles.
Stacks and Queues
- Implement Stack using List / Linked List
- Implement Queue using List / Deque
- Design a Stack with getMin() in O(1)
- Implement a Circular Queue
- Implement a Queue using Two Stacks
Trees
- Implement Binary Tree and Traversals (Inorder, Preorder, Postorder)
- Check if a Binary Tree is Balanced
- Find Height and Diameter of Binary Tree
- Lowest Common Ancestor (LCA)
- Level Order Traversal (BFS)
- Convert Binary Tree to Doubly Linked List
Graphs
- Implement Graph using Adjacency List
- DFS and BFS Traversals
- Detect Cycle in a Graph
- Check if Graph is Bipartite
- Topological Sort
- Number of Islands (DFS based)
Heaps and Priority Queues
- Implement MinHeap and MaxHeap
- K Largest Elements in an Array
- Merge K Sorted Lists
- Find Median from Data Stream
Use Python’s heapq module or implement your own heap logic.
Tries (Prefix Trees)
- Insert and Search Words in Trie
- Auto-suggestion Feature
- Word Search in a 2D Grid using Trie
Hashing and Sliding Window
- Longest Substring with K Unique Characters
- Count Anagrams in a String
- Maximum Sum Subarray of Size K
- Find All Subarrays with Sum = K
These problems often appear in companies like Google, Amazon, and Microsoft.
Final Tips, Daily Practice Plan & Best Resources
You’ve got the roadmap. You’ve got the practice sets. Now let’s bring it all together with a daily coding strategy and hand-picked resources to fast-track your Python mastery.
Final Tips to Master Python Coding Rounds
- Start small, stay consistent – Don’t overwhelm yourself. Do 2–3 problems a day, but every single day.
- Focus on patterns, not just solutions – Understand “why” a solution works.
- Learn to debug like a pro – Use print statements or pdb to trace logic.
- Write clean, modular code – Follow PEP8 standards and add comments.
- Re-solve problems without looking at answers – That’s how real mastery is built.
Top Resources to Practice Python Programming
Platforms for Practice:
- LeetCode – Best for interview-level problems
- HackerRank – For basic to intermediate level
- GeeksForGeeks – All levels + theory
- Codeforces – Competitive programming
- Project Euler – Math-based Python practice
YouTube Channels:
- Krish Naik
- Tech with Tim
- Codebasics
- Neetcode
- Programming with Mosh
Conclusion
Mastering Python isn't just about writing syntax-correct code—it's about building the ability to solve real-world problems, crack coding interviews, and think like a developer. By practicing these curated sets of Python programs, from the most basic to the highly advanced, you're not just learning to code—you're developing logical thinking, improving your problem-solving skills, and preparing yourself for technical rounds at top companies. Start small with the basics, challenge yourself with intermediate problems, and gradually push your boundaries with advanced algorithmic questions.
The key is consistency and a curious mind. Make problem-solving a daily habit, analyze your mistakes, revisit concepts when needed, and never shy away from tough problems—they’re the ones that shape you the most. With dedication and smart practice, you’ll not only gain confidence in Python but also position yourself for high-growth career opportunities in tech.
So keep coding, keep exploring, and remember—every great developer was once a beginner who didn’t quit.