In the modern world, where data is an essential asset for any organization, the ability to effectively query databases is crucial.To retrieve data commands are used. SQL, or Structured Query Language, serves as the backbone of relational databases, enabling users to create, modify, and query data efficiently. In this comprehensive guide, we will explore SQL commands in detail, delve into advanced topics, and provide practical examples to enhance your SQL querying skills.
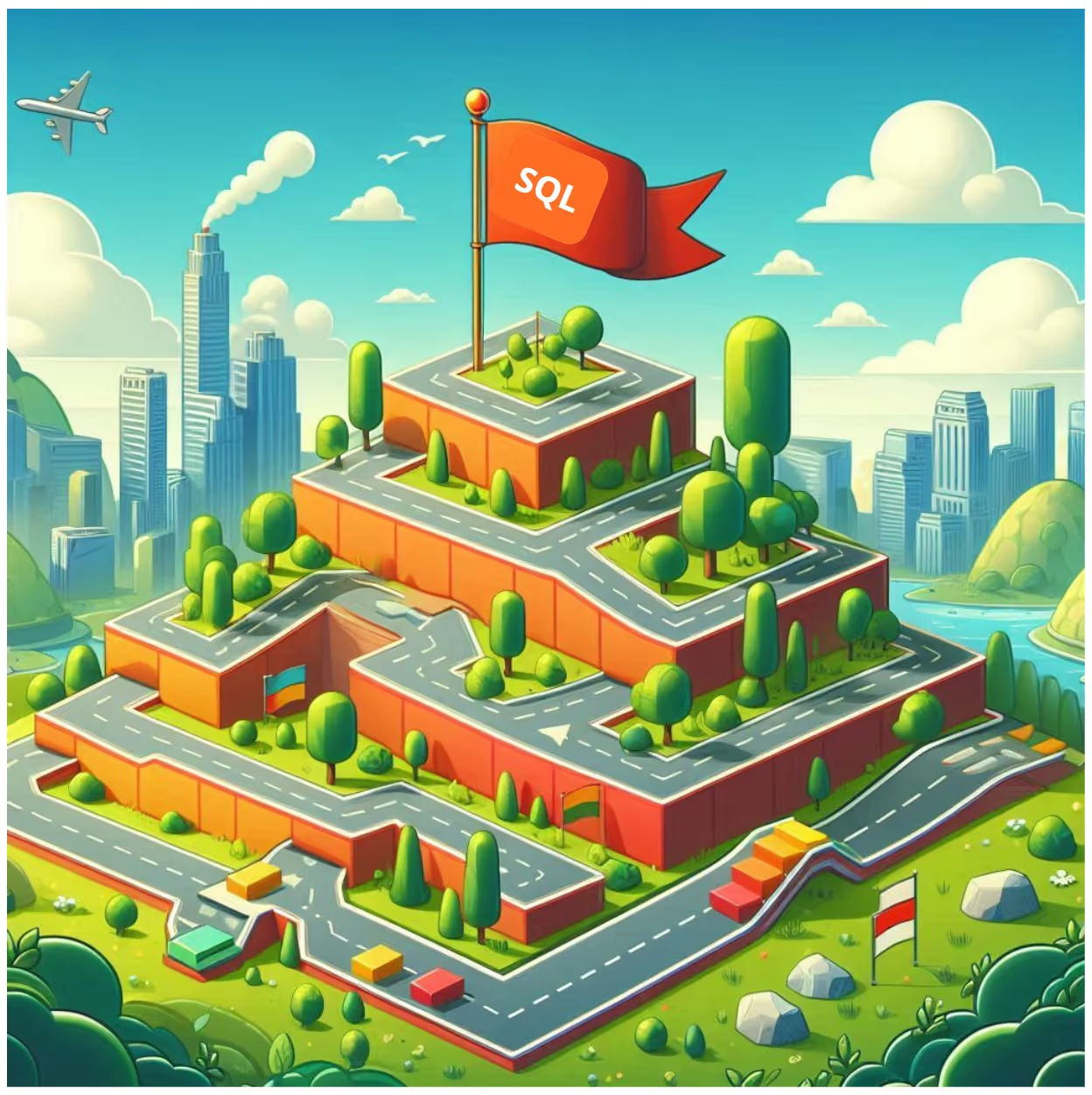
1. Introduction to SQL
SQL, or Structured Query Language, is the standard programming language used for managing and manipulating relational databases. With SQL, users can perform various operations, including data retrieval, insertion, updating, deletion, and even database schema modification. The primary goal of SQL is to provide a straightforward and efficient way to interact with data stored in relational databases, allowing users to make informed decisions based on accurate information.
2. Understanding SQL Basic Commands
SQL commands can be categorized into several types based on their functionality. Understanding these basic commands is essential for effective database querying.
SELECT Command
The SELECT command is the most fundamental SQL command. To retrieve data commands are used on one or more tables in a database.
Basic Syntax:
SELECT column1, column2, ...
FROM table_name
WHERE condition;
Example:
SELECT first_name, last_name
FROM employees
WHERE department = 'Sales';
This command retrieves the first and last names of employees working in the Sales department.
Advanced Usage:
Using Aliases: Aliases allow you to rename columns in your output for better readability.
SELECT first_name AS "First Name", last_name AS "Last Name"
FROM employees;
Sorting Results: The ORDER BY clause sorts the results based on specified columns.
SELECT * FROM employees
ORDER BY hire_date DESC;
Limiting Results: The LIMIT clause restricts the number of records returned.
SELECT * FROM employees
LIMIT 5;
INSERT Command
The INSERT command is used to add new records to a table.
Basic Syntax:
INSERT INTO table_name (column1, column2, ...)
VALUES (value1, value2, ...);
Example:
INSERT INTO employees (first_name, last_name, department)
VALUES ('John', 'Doe', 'Marketing');
This command adds a new employee named John Doe to the Marketing department.
Advanced Usage:
Inserting Multiple Records:
INSERT INTO employees (first_name, last_name, department) VALUES
('Alice', 'Smith', 'HR'),
('Bob', 'Johnson', 'IT');
Inserting Data from Another Table: You can insert data from one table into another.
INSERT INTO employees_backup (first_name, last_name, department)
SELECT first_name, last_name, department
FROM employees
WHERE hire_date < '2020-01-01';
UPDATE Command
The UPDATE command modifies existing records in a table.
Basic Syntax:
UPDATE table_name
SET column1 = value1, column2 = value2, ...
WHERE condition;
Example:
UPDATE employees
SET department = 'Sales'
WHERE first_name = 'John' AND last_name = 'Doe';
Here, John Doe's department is updated to Sales.
Advanced Usage:
Updating Multiple Columns:
UPDATE employees
SET department = 'Sales', hire_date = '2022-01-01'
WHERE first_name = 'John' AND last_name = 'Doe';
Conditional Updates:
UPDATE employees
SET department = 'Contract'
WHERE department = 'Internship';
DELETE Command
The DELETE command removes records from a table.
Basic Syntax:
DELETE FROM table_name
WHERE condition;
Example:
DELETE FROM employees
WHERE first_name = 'John' AND last_name = 'Doe';
This command deletes John Doe's record from the employees table.
Advanced Usage:
Deleting All Records: If you want to delete all records, use a DELETE command without a WHERE clause. However, be cautious with this operation.
DELETE FROM employees; -- Deletes all records
Using JOIN in DELETE: You can delete records from a table based on conditions from another table.
DELETE e
FROM employees e
JOIN departments d ON e.department = d.department_name
WHERE d.location = 'Remote';
3. Data Definition Commands
Data definition commands are used to define and modify the structure of a database. They include commands like CREATE TABLE, ALTER TABLE, and DROP TABLE.
CREATE TABLE Command
The CREATE TABLE command is used to create a new table in the database.
Basic Syntax:
CREATE TABLE table_name (
column1 datatype,
column2 datatype,
...
);
Example:
CREATE TABLE employees (
employee_id INT PRIMARY KEY,
first_name VARCHAR(50),
last_name VARCHAR(50),
department VARCHAR(50),
hire_date DATE
);
In this example, a new table named employees is created with four columns: employee_id, first_name, last_name, and department.
Advanced Usage:
Adding Constraints: Constraints enforce rules on the data in a table.
CREATE TABLE employees (
employee_id INT PRIMARY KEY,
first_name VARCHAR(50) NOT NULL,
last_name VARCHAR(50) NOT NULL,
department VARCHAR(50) CHECK (department IN ('HR', 'IT', 'Finance')),
hire_date DATE DEFAULT CURRENT_DATE
);
ALTER TABLE Command
The ALTER TABLE command modifies an existing table, allowing you to add, delete, or change columns.
Basic Syntax:
ALTER TABLE table_name
ADD column_name datatype;
Example:
ALTER TABLE employees
ADD email VARCHAR(100);
This command adds a new column called email to the employees table.
Advanced Usage:
Dropping a Column:
ALTER TABLE employees
DROP COLUMN email;
Changing a Column Data Type:
ALTER TABLE employees
ALTER COLUMN hire_date SET DATA TYPE TIMESTAMP;
DROP TABLE Command
The DROP TABLE command deletes an entire table and its data from the database.
Basic Syntax:
DROP TABLE table_name;
Example:
DROP TABLE employees;
This command will permanently remove the employees table from the database.
Advanced Usage:
Conditional Drop: To avoid errors if the table doesn’t exist, you can use:
DROP TABLE IF EXISTS employees;
4. Data Control Commands
Data control commands are used to control access to data within the database. These commands include GRANT and REVOKE, which manage user permissions.
GRANT Command
The GRANT command assigns privileges to users.
Example:
GRANT SELECT, INSERT ON employees TO user_name;
This command grants the user permission to select and insert data in the employees table.
REVOKE Command
The REVOKE command removes user privileges.
Example:
REVOKE INSERT ON employees FROM user_name;
This command removes the user's permission to insert data into the employees table.
5. Advanced SQL Concepts
To further enhance your SQL skills, let’s explore some advanced SQL concepts that will allow you to perform more complex queries and operations.
Joins
Joins are used to combine records from two or more tables based on related columns.
INNER JOIN: Returns records with matching values in both tables.
SELECT e.first_name, e.last_name, d.department_name
FROM employees e
INNER JOIN departments d ON e.department = d.id;
LEFT JOIN: Returns all records from the left table and matched records from the right table.
SELECT e.first_name, e.last_name, d.department_name
FROM employees e
LEFT JOIN departments d ON e.department = d.id;
RIGHT JOIN: Returns all records from the right table and matched records from the left table.
SELECT e.first_name, e.last_name, d.department_name
FROM employees e
RIGHT JOIN departments d ON e.department = d.id;
FULL OUTER JOIN: Returns records when there is a match in either left or right table records.
SELECT e.first_name, e.last_name, d.department_name
FROM employees e
FULL OUTER JOIN departments d ON e.department = d.id;
Subqueries
Subqueries are nested queries that allow you to perform operations based on the results of another query.
Example:
SELECT first_name, last_name
FROM employees
WHERE department IN (SELECT department_name FROM departments WHERE location = 'Remote');
This command retrieves the names of employees whose departments are located remotely.
Views
Views are virtual tables that provide a way to simplify complex queries. They can encapsulate complex SELECT statements and be used as a regular table.
Creating a View:
CREATE VIEW employee_view AS
SELECT first_name, last_name, department
FROM employees;
Using a View:
SELECT * FROM employee_view;
Indexes
Indexes are used to speed up the retrieval of data from tables. They can significantly improve query performance.
Creating an Index:
CREATE INDEX idx_department ON employees(department);
Using Indexes: When querying the employees table, the database will use the index to locate records faster.
Transactions
Transactions ensure that a series of SQL operations are executed in a way that maintains data integrity. They follow the ACID properties: Atomicity, Consistency, Isolation, and Durability.
Using Transactions:
BEGIN;
INSERT INTO employees (first_name, last_name, department)
VALUES ('Jane', 'Doe', 'Marketing');
UPDATE employees
SET department = 'Sales'
WHERE first_name = 'John';
COMMIT; -- Saves all changes
If an error occurs, you can use ROLLBACK to undo changes made in the transaction.
6. Practical Examples of SQL Basic Commands
Let’s apply what we’ve learned through a series of practical examples using a sample database of employees and departments.
Creating the Employees Table
To start, we’ll create an employees table using the CREATE TABLE command:
CREATE TABLE employees (
employee_id INT PRIMARY KEY,
first_name VARCHAR(50),
last_name VARCHAR(50),
department VARCHAR(50),
hire_date DATE,
email VARCHAR(100)
);
Creating the Departments Table
Next, we’ll create a departments table:
CREATE TABLE departments (
id INT PRIMARY KEY,
department_name VARCHAR(50),
location VARCHAR(50)
);
Inserting Records
Next, we can insert several employee and department records:
INSERT INTO departments (id, department_name, location)
VALUES
(1, 'HR', 'Head Office'),
(2, 'IT', 'Remote'),
(3, 'Marketing', 'Head Office');
INSERT INTO employees (employee_id, first_name, last_name, department, hire_date, email)
VALUES
(1, 'Alice', 'Smith', 'HR', '2020-01-15', 'alice@example.com'),
(2, 'Bob', 'Johnson', 'IT', '2019-03-22', 'bob@example.com'),
(3, 'Charlie', 'Brown', 'Finance', '2021-07-30', 'charlie@example.com');
Querying the Data
To retrieve all data from both tables, we’ll use the SELECT command:
SELECT e.first_name, e.last_name, d.department_name
FROM employees e
INNER JOIN departments d ON e.department = d.department_name;
Updating a Record
Suppose we need to update Charlie Brown’s department:
UPDATE employees
SET department = 'IT'
WHERE employee_id = 3;
Deleting a Record
If we want to remove Alice Smith from the table:
DELETE FROM employees
WHERE employee_id = 1;
Altering the Table
Let’s add a new column for the employee’s phone number:
ALTER TABLE employees
ADD phone_number VARCHAR(15);
Creating a View
To simplify reporting, we can create a view:
CREATE VIEW employee_info AS
SELECT first_name, last_name, department
FROM employees;
Using the View
We can now query the view:
SELECT * FROM employee_info;
Implementing Transactions
When updating multiple records, we can use transactions to ensure data integrity:
BEGIN;
UPDATE employees
SET department = 'Sales'
WHERE department = 'Marketing';
UPDATE departments
SET location = 'Branch Office'
WHERE department_name = 'Sales';
COMMIT;
7. Best Practices for Using SQL Commands
To maximize the effectiveness of your SQL querying, consider the following best practices:
- Use Descriptive Table and Column Names: Choose names that clearly indicate the content and purpose. For instance, use employee_email instead of just email to avoid ambiguity.
- Limit the Use of SELECT : Instead of using SELECT *, specify the columns you need. This practice improves performance and makes your queries clearer:
SELECT first_name, last_name FROM employees;
- Use WHERE Clauses Wisely: Always use WHERE clauses in your DELETE and UPDATE commands to avoid unintended data loss:
DELETE FROM employees WHERE employee_id = 1; - Test Your Queries: Before running commands that alter data (INSERT, UPDATE, DELETE), run SELECT statements to ensure you’re targeting the right data.
- Keep Security in Mind: Use data control commands to manage user access. Ensure only authorized users have the ability to modify critical data.
- Optimize Queries: Use indexes for frequently queried columns to speed up data retrieval.
- Document Your SQL Code: Comment on your SQL code for future reference, making it easier to understand and maintain.
8. Conclusion
Mastering SQL all commands is essential for anyone who works with databases. By understanding and effectively using commands such as SELECT, INSERT, UPDATE, DELETE, and CREATE TABLE, you can perform a wide variety of data operations. Furthermore, diving into advanced topics like joins, subqueries, views, indexes, and transactions will empower you to handle complex queries and operations with ease.
As you continue your journey into the world of SQL, remember that practice is key. Practicing sql all commands, experiment with these commands in a safe environment to build your confidence and skills. Whether you’re a developer, a data analyst, or a business professional, mastering SQL will enable you to leverage the full potential of your data, make informed decisions, and contribute significantly to your organization’s success. Practice by doing varieties of SQL projects.
With dedication and practice, you’ll find yourself navigating SQL databases like a seasoned professional. Embrace the challenge, and enjoy the journey!
Ready to transform your AI career? Join our expert-led courses at SkillCamper today and start your journey to success. Sign up now to gain in-demand skills from industry professionals.
If you're a beginner, take the first step toward mastering Python! Check out this Fullstack Generative AI course to get started with the basics and advance to complex topics at your own pace.
To stay updated with latest trends and technologies, to prepare specifically for interviews, make sure to read our detailed blogs:
How to Become a Data Analyst: A Step-by-Step Guide
How Business Intelligence Can Transform Your Business Operations