Introduction
Ever wondered how Python stores all the different kinds of information you throw at it? From numbers to words, lists to dictionaries, Python's got a bunch of different ways to handle data, and these are called datatypes. Think of datatypes as different containers that hold specific kinds of data, each with its own quirks and superpowers.
In this article, we’re going to break down Python’s most common datatypes—like integers, strings, lists, and more—so you can start understanding how Python works under the hood. Whether you're just getting started or need a quick refresher, this guide will help you make sense of Python’s core data handling tools. Let's dive in!
What are Python data types?
Data types are basically the categories that tell Python what kind of value you're working with. Whether it's a number, some text, or a more complex structure like a list, each data type has its own way of behaving. Knowing about data types helps Python understand how to handle and store the data you give it, making sure everything runs smoothly. From basic stuff like numbers to more advanced things like dictionaries, each type serves a different purpose in your code.
Before we get further into the various python data types, here’s a list of some words you might be hearing often, and what they mean.
Mutability
Mutability refers to whether an object’s value can be changed after it’s created. In Python, some data types are mutable, meaning you can modify them in place, while others are immutable, meaning once they’re created, their value cannot be changed.
Orderability
Orderability refers to whether or not a data type supports is stored in a set sequence
Iterability
Iterability refers to the ability of an object to be looped over, or iterated through, one element at a time. In other words, an iterable is any object that can return its elements one by one, allowing you to use loops like for loops to process each element sequentially.

The above image shows how an iterable is iterated with an iterator.
Hashability
Hashability refers to whether an object of a certain data type is hashable i.e. if it can be used as a key in a dictionary or as an element in a set. In short, a hashable data type can be assigned a unique “hash” value which does not change in its lifetime.
This requires an object to be immutable. So, if you were wondering why objects were immutable in the first place, it is for this reason.
A mutable item has the benefit of being changeable, while an immutable item has better data integrity, faster and more efficient data retrieval and can be uniquely identified.
Setting a data type
By default, Python is a high-level data language. This means that when you write code in it, you don’t have to worry about its architecture, memory management, or structure of code. As a result, the data type is automatically set when you simply assign a value to a variable.

However, you can also specify the data type if you like
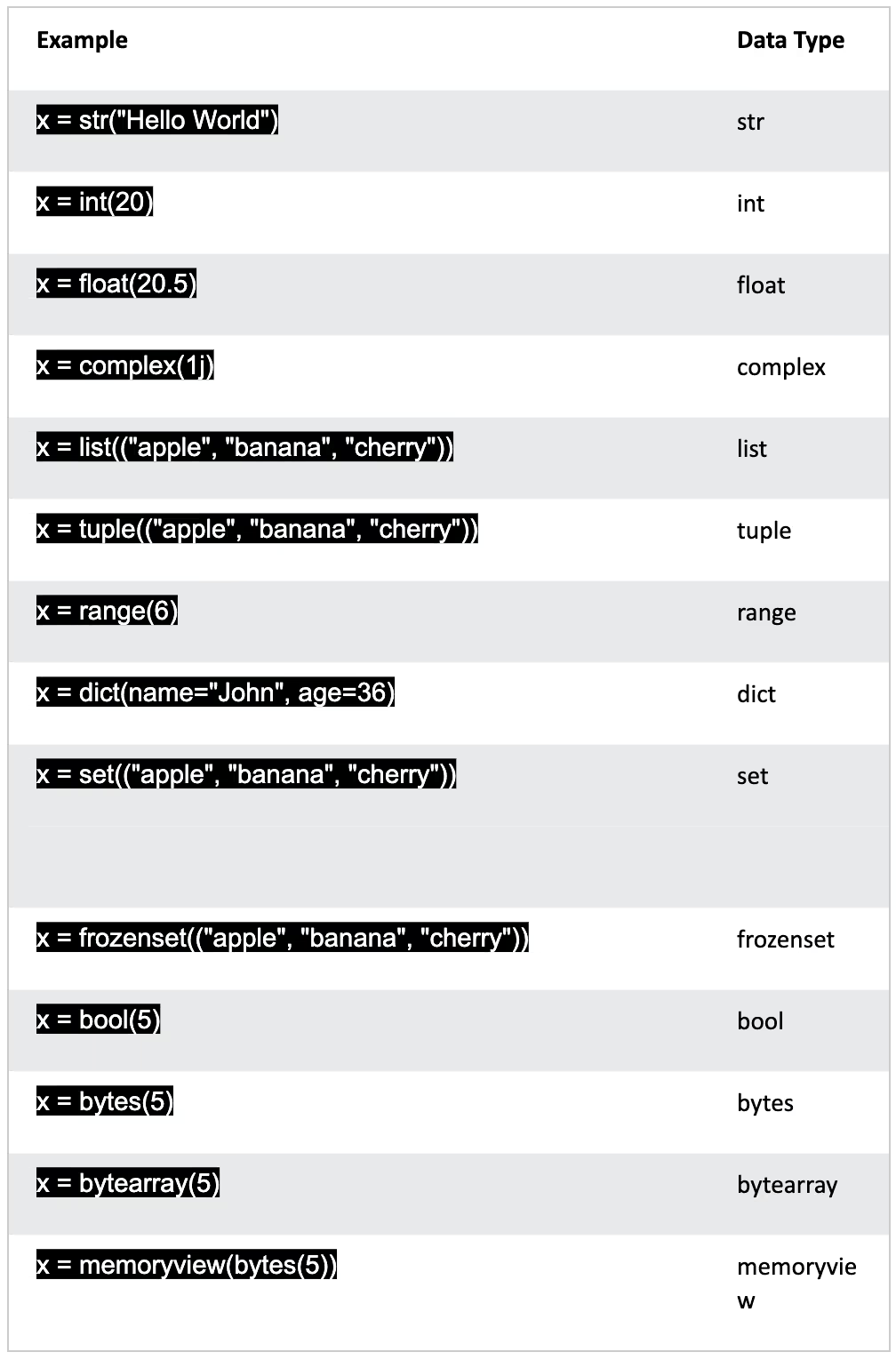
Categories

Illustrated in this chart is a comprehensive list of the built-in data types in Python.
1. Numeric
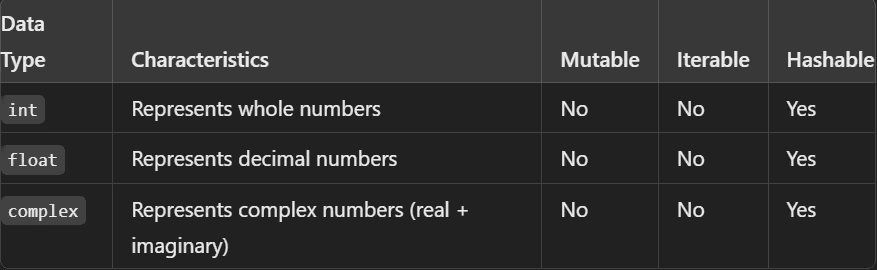
Numeric data types in python are used to represent numbers. Here are the 3 numeric data types you’ll encounter
In Python, numerical data types are used to represent numbers. Here are the main numerical data types you'll encounter:
- int (Integer): Represents whole numbers, both positive and negative, without any decimal points. For example, 5, -3, and 42 are integers.
Syntax:
my_int = 10 # Positive integer
my_negative_int = -5 # Negative integer
- float (Floating-point): Represents numbers that have a decimal point. They can be positive or negative. For example, 3.14, -0.001, and 2.71828 are floats.
Syntax:
my_float = 3.14 # Positive float
my_negative_float = -2.718 # Negative float
- complex (Complex): Represents numbers that have a real part and an imaginary part. The imaginary part is indicated with a j (or J). For example, 3 + 4j is a complex number with a real part of 3 and an imaginary part of 4.
Syntax:
my_complex = 2 + 3j # Complex number with real part 2 and imaginary part 3j
Numeric data functions
Here are some numeric data functions in Python

2. Text
In Python, the text data type is primarily represented by the str (string) type. A string is a sequence of characters enclosed in quotes (single, double, or triple quotes). Here’s a brief overview of the str type:
Characteristics of str:
- Immutable: Once a string is created, it cannot be changed. Operations that seem to modify a string actually create a new string.
- Iterable: You can loop through a string, accessing each character individually.
- Hashable: Strings can be used as dictionary keys or set elements, provided that the string itself is not modified.
Here are some of the commonly used built-in functions for strings
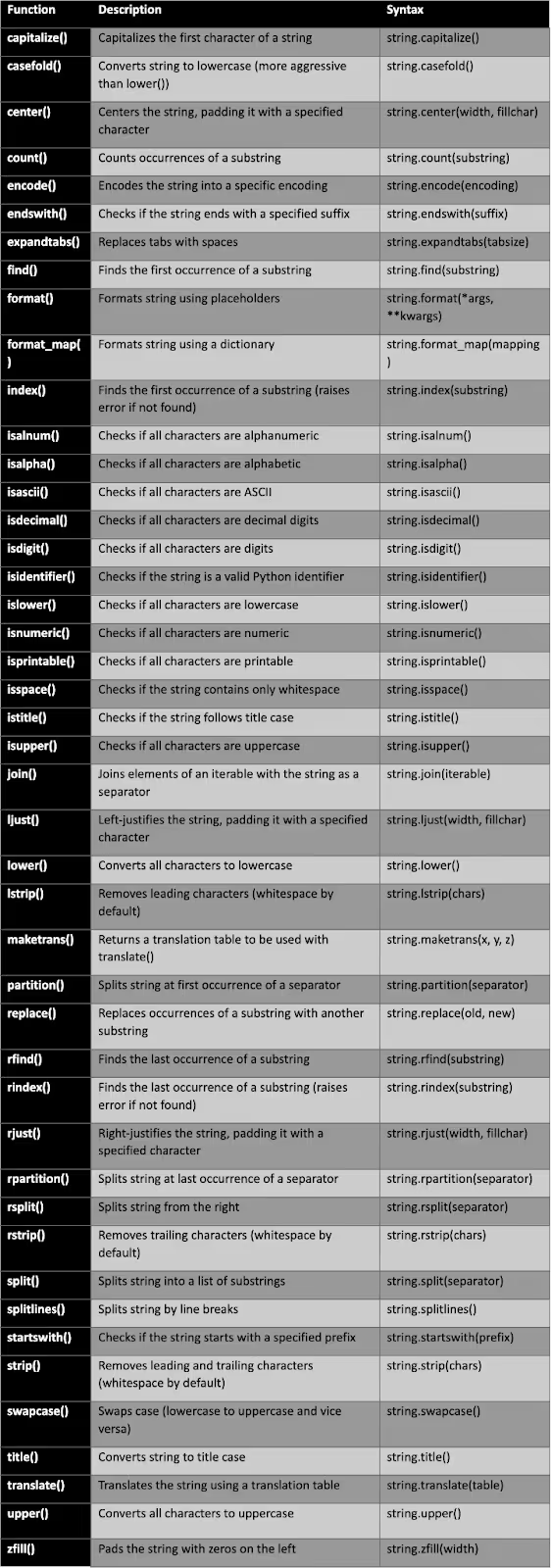
3. Sequence
Sequence data types are types that represent ordered collections of items. These items can be of any type, and sequences allow you to access them using indices.
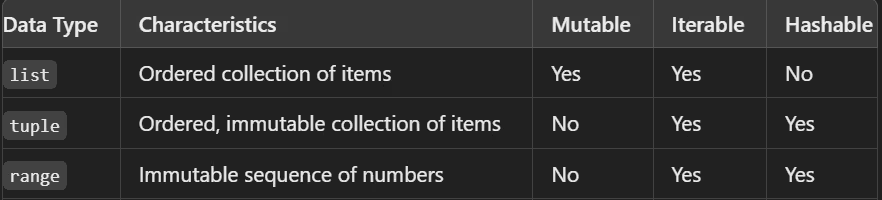
i) List
- A list is an ordered, mutable collection of items.
- A list can contain elements of different types, including other sequences.
- A list uses square brackets.
- E.g
my_list = [1, 2, 3, 'four', [5, 6]]
ii) Tuple
- A tuple is also an ordered collection of items, but is immutable.
- Tuples can contain elements of different types.
- Tuples use round brackets.
- E.g
my_tuple = (1, 2, 3, 'four', (5, 6))
iii) Range
- A range is an immutable sequence of numbers, commonly used in loops to iterate over a sequence of integers.
- A range object can be created using the range() function.
- Syntax:some text
- One argument: r = range(5) (Represents numbers from 0 to 4)
- Two arguments: r = range(2, 5) (Represents numbers from 2 to 4)
- Three arguments: r = range(1, 10, 2) (Represents numbers from 1 to 9 with a step of 2)
- The range function in Python, when called, creates a range object, which is a special type of iterable that represents a sequence of numbers. If you try to print or directly view a range object without iteration, it will show you its representation rather than the sequence of numbers it generates.

Common Sequence Functions/Methods

Here’s the updated table of common sequence functions and methods in Python, including the list, tuple, and range data types. String-specific methods are excluded.
List-Specific Methods

Tuple-Specific Operations
- Tuples are immutable, so methods that modify elements (like append() or remove()) do not apply to tuples. However, tuples support most common sequence operations, including:some text
- count()
- index()
- Slicing, concatenation, and repetition
Range-Specific Properties

A range is an immutable sequence of numbers commonly used in loops. It does not support methods that modify content but can be sliced and iterated over.
4. Mapping
In Python, a mapping type refers to a data structure that stores data in key-value pairs. A mapping type allows you to associate a unique key with a value, enabling fast lookups, insertions, and deletions based on the key. The only built-in mapping data type in Python is dict(dictionary).

Dictionary
- Stores data as key-value pairs
- Each key in the dictionary must be unique
- Dictionaries themselves are mutable. You can change, add or remove key-value pairs after creation.
- Order: Prior to Python 3.7, dictionaries were unordered. This means that the insertion order of keys is not guaranteed. From Python 3.7 onwards, dictionaries maintain the order of insertion.
- Syntaxsome text
- Creating a dictionary:
my_dict = {
"name": "Alice",
"age": 25,
"city": "New York"
}
- Accessing values by key:
print(my_dict["name"]) # Output: Alice
- Modifying a value:
my_dict["age"] = 26
5. Set
A set in python is an unordered collection of unique elements.
- A set does not maintain an order of elements. You cannot rely on the order in which items are inserted
- Unique elements: Sets automatically eliminate duplicates
- Sets are mutable. You can add and remove elements after a set is created
- No indexing/slicing: Because sets are unordered, they do not support indexing or slicing like lists or tuples.
- Only immutable items can be added to a set
- Syntax: A set can be created in 2 wayssome text
- By using the set() function with lists or tuples
thisset = set(("apple", "banana", "cherry")) # from a Tuple
- By using the set() function with lists or tuples
thisset = set(["apple", "banana", "cherry"]) # from a List
- By declaring a set
thisset = {"apple", "banana", "cherry"}
print(thisset)
Set Syntax and Methods

Set Operations

6. Frozenset
The frozenset data type is simply an immutable version of the set data type. This means you cannot add, remove or edit elements after creating a frozenset data type.
7. Binary
Think of binary data as a way of representing information using only two symbols: 0 and 1. Computers use this binary system because they work with electrical signals that can be either on (1) or off (0). The 3 built-in binary data types in Python are:
- Bytes
- Bytearray
- Memoryview
Let us look into these in detail:
i) Bytes
Bytes are a sequence of numbers where each number is between 0 and 255. Because there are 8 bits in a byte, you can have 2^8 or 256 different combinations of 0s and 1s. Each number is like a tiny piece of information. Bytes are used for handling binary data, such as reading/writing files, network communication, or encoding/decoding data. Bytes are immutable.
my_bytes = b'hello' # Creates a sequence of bytes that represents the word 'hello'
print(my_bytes) # Output: b'hello'
ii) Bytearray
Bytearrays are similar to bytes, but are mutable. We use this to modify binary data in place, such as when processing binary files or network protocols.
my_bytearray = bytearray(b'hello') # Create a mutable sequence of bytes
my_bytearray[0] = 72 # Change the first byte
print(my_bytearray) # Output: bytearray(b'Hello')
iii) Memoryview
A memoryview type to create a “view” of memory containing binary data. It doesn’t store the data itself but provides a view into the memory where the data is stored. These are handy for efficiently manipulating large amounts of data without copying it. Memory views are typically used in advanced scenarios where direct memory manipulation is required, such as in high-performance applications.
data = bytearray(b'hello')
view = memoryview(data) # Create a view of the data
print(view[0]) # Output: 104 (ASCII value for 'h')
view[0] = 72 # Modify the first byte through the view
print(data) # Output: bytearray(b'Hello')
8. None
None is a special constant in Python that represents the absence of a value or a null value.
The none data type is a singleton. That means there is only one possible instance of None in a python program.
Checking a data type
Checking data types in Python is essential for ensuring that variables and values are of the expected type before performing operations on them. In Python, the type() function is used, which outputs the data type of a variable.
x = 10
print(type(x)) # Output: <class 'int'>
y = "Hello"
print(type(y)) # Output: <class 'str'>
Conclusion
So there you have it! Python's data types might seem like a lot to take in at first, but they're pretty straightforward once you get the hang of them. From the basic types like integers and strings to more advanced ones like bytes, bytearrays and memoryviews, each type has its own special role.
Remember, choosing the right data type is crucial for writing efficient and bug-free code. Whether you're dealing with numbers, text, collections of items, or even binary data, Python has got you covered with a rich set of tools to make your life easier.
So, next time you're coding, take a moment to think about what kind of data you're working with and pick the type that fits best. It’ll make your code cleaner, faster, and just plain better. If you’re interested in learning Python, check out our free python course.
Resources:
Python data types – Official documentation
Understanding data types in Python – A comprehensive guide
Blog Title (H1)
Data types in Python
Objective
Illustrate the various data types in python with syntax
Target Audience
(Eg: Tech enthusiasts, business professionals, AI researchers, general audience who just want to familiarize themselves with the concepts, Chief Data Officer, CTOs, CIOs and other executives who manage data teams etc.)
Python novices
Awareness Level
Novice (no idea about data science), beginner (just starting their Data Science journey), practitioner (intermediate and expert)
beginner
Persona
(Ignore this for now, I will share a list of personas with you briefly)
Primary keyword
Python Data Types
Secondary keyword(s)
python data
various data types in python
Meta title
(same as H1)
Data types in Python
Meta description
(appears in google search, must contain primary keyword)
Discover the essential Python data types—integers, strings, lists, dictionaries, and more. Learn how to handle and manipulate data efficiently in Python with this beginner-friendly guide.
2 line blog summary
(appears on the blog category page)
Discover the essential Python data types—integers, strings, lists, dictionaries, and more. Learn how to handle and manipulate data efficiently in Python with this beginner-friendly guide.
Word Count
Goal/ Objective
Eg: The blog aims to serve as a comprehensive resource for understanding the python data types
Call To Action (CTA)
(link to one of our courses, free courses organically, no force-fitting)
If you’re interested in learning Python, check out our free python course.
*Formatting guideline
Please tag the H1, H2, H3, H4 and so on in the google doc itself. Just look at the font styles and instead of ‘Normal text’ choose Heading 2, Heading 3, Heading 4 etc. It makes publishing easier.
*Content Guidelines
1) Use the primary keyword in the title
2) Give a Table of Contents where possible
2) Use it 2-3 times in the body of the blog including the conclusion. If it feels unnatural or force-fitted you can do it only twice. The third time you can use synonyms as well. Eg components of data science can be building blocks of data science or something similar.
3) Try and end with a call to action (CTA) that asks the reader if they want to learn more, try their hand at xyz tool or programming language, want to become a data scientist etc - to sign up for one of our courses all of which are listed on skillcamper.com and link to them.
4) Also now that you’ve written several blogs around the same subject - eg Data Science, you can organically link to it in your subsequent piece on a similar subject or to pieces others have done. Eg How to write basic SQL queries for data retrieval can link to introduction to SQL and vice versa
Comment:
Interlink the keywords to a suitable link